About the Class
Intro
- Analog vs digital
- analog has continuous values vs digital, which has only steps of values
- how do we do that?
- Through transistors, little electronic switches that can open or close a circuit
- Through that we can build AND OR gates
- These AND OR gates, combined with transistors to store states, this can scale
- -> Microchips
- PCBs vs ICs
- PCB -> laser etched circuit board
- IC -> little black boxes -> miniaturization
- Microcontroller -> mostly single-purpose, programmable
- Microprocessors -> able to run OS, more multi-purpose, programmable, multi-tasking
Compiling
- 1MHz -> number of operations per second, number of inputs & output to change per second
- I2C, SPI protocols in dedicated parts of the chip
- Compiling is the process of converting our program (that we humans understand) into machine code (that the chip understands)
- Steps are:
- Pre-processor adds extra libraries to the code and checks for integrity
- Dependencies are resolved
- Compiling locally
- Uploading to board
- Flashing the board via the bootloader (the Bootloader manages the root on the board to receive and store programs to execute)
Programming
- Most Microcontrollers use C++ for programming
- There is an emerging system for writing Python for MCs, but it is not fully fleshed out
- Although it seems like a shortcut to programming for microcontrollers, but using python to learn will cause problems later on
- -> Always start from a programming flowchart
- To save states -> Variables: Boolean (true, false), floating-point (constantliy changingvalues), integer, list or array (list of values), etc.
- Loops: close (some feedback to the loop to verify states) , open (no feedback back to loop)
Spirulina Temp Sensor
As for the homework, I programmed a simple temperature sensor for a jar of spirulina (which only grows in moderately warm temperatures), featuring a DT11 sensor and the Adafruit LED strip. After pressing a button, the temperature is measured by the DT11 and a visual feedback can be observed on the LED strip (red, blinking = too hot; green = optimal range; blue, blinking = too cold).
Testing the final setup on my terrace.
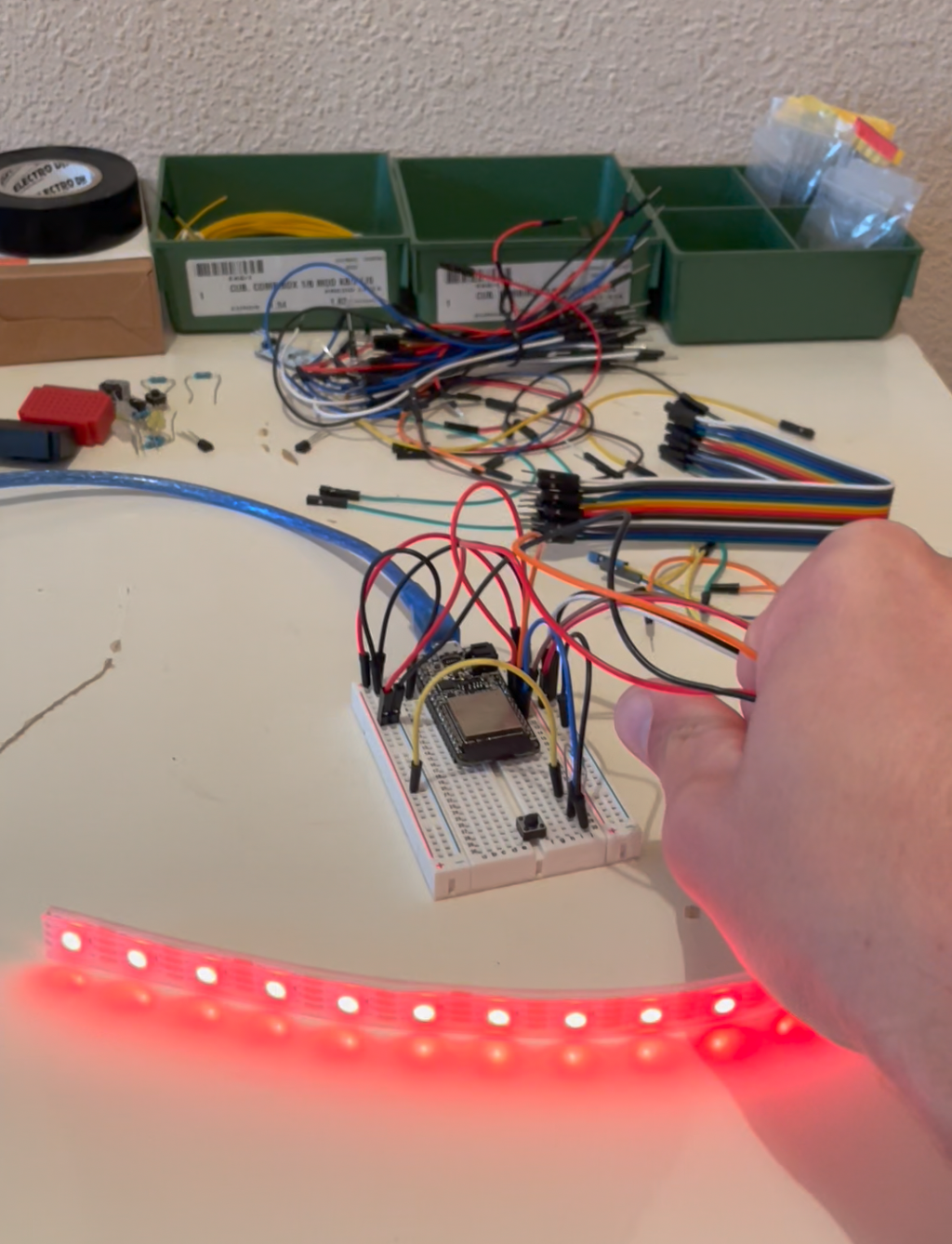
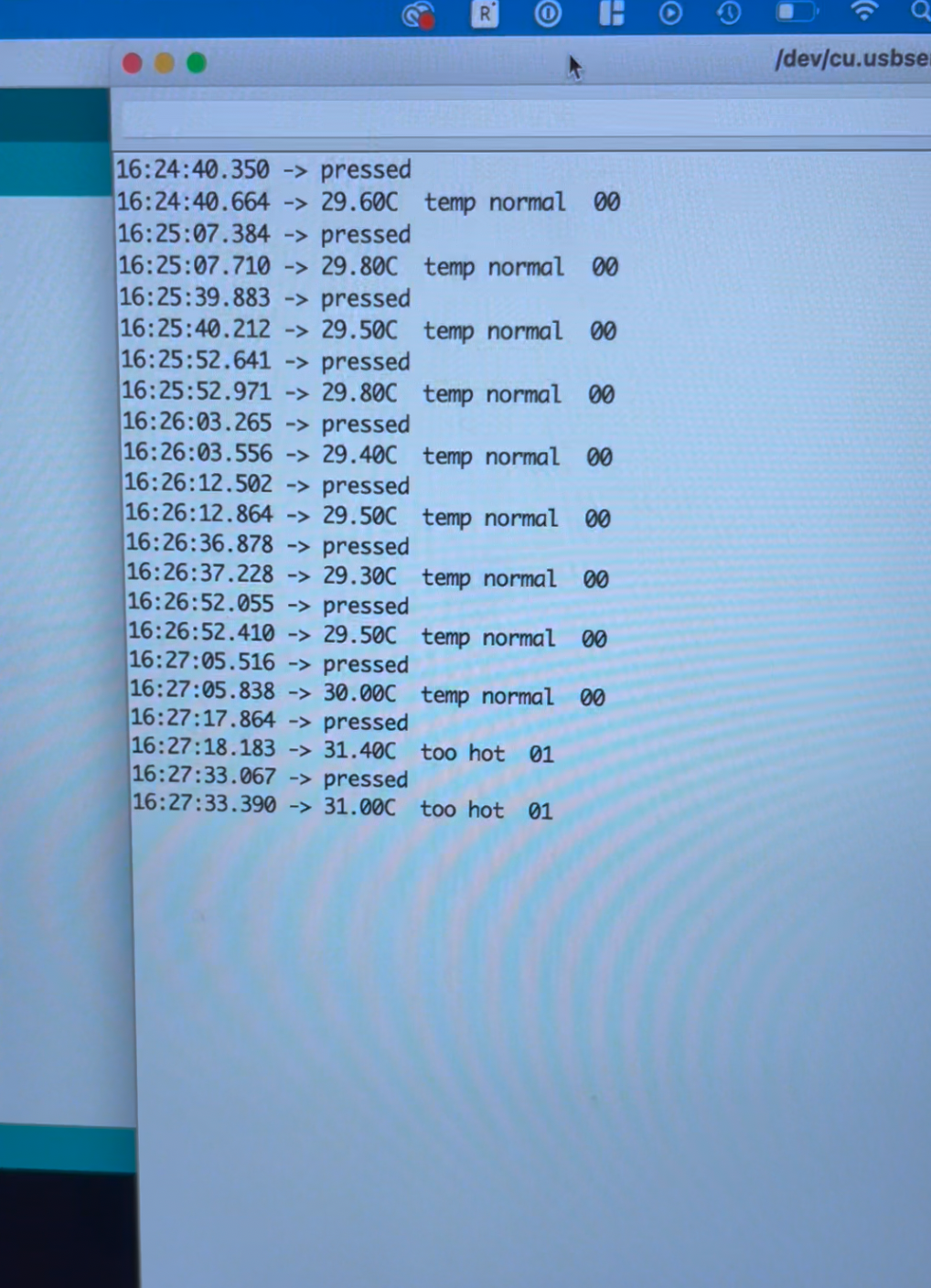
Debugging the visual feedback: After heating the DT11 close to body temperature, the LEDs flash bright red. On the right, the serial output can be see.
Further Links
architectures
von Neumann, Harvard, bugs
RISC, CISC
microprocessor, microcontroller
multi-core
GPU, TPU
FPGA, TinyFPGA, IceStorm
,
Migen
spatial
memory
registers (instructions)
SRAM (fast)
DRAM (big)
EEPROM (non-volatile)
FLASH (programs, strings)
fuse (configuration)
peripherals
ports
A/D
comparator
D/A
timer/counter/PWM
USART
USB
math
crypto
...
word size
8
16
32
64
families
LOGIC
Megaprocessor
8051
PIC
MSP
AVR
• 6-100 pin packages
ATtiny10
• SOT23-6
ATtiny45V, ATtiny44A
• prior
ATtiny412, ATtiny1614, ATtiny3216
• 1-series
• 8 bit, 1.8-5.5V, 20 MHz
• single-cycle global instructions
• simple peripheral register access
• low pin-count packages
• one-pin serial programming
ATtiny1624
• 2-series
• programmable gain amplifier
AVR128DB32
• 24 MHz
• analog signal conditioning
• level shifting
• hardware multiplier
DD
• coming
ARM
D11C, D11D, D21E, D51
• 32 bit, 1.6-3.6V
• faster clocks, more complex clock distribution and synchronization
• more powerful peripherals, more complex register access and libraries
• diverse family, larger packages
• standard in-circuit debugging
Xtensa
ESP8266, ESP32
• integrated RF, networking
RISC-V
• open architecture
PSoC, xCORE, Propeller, Lattice, NVIDIA
• parallel
vendors
Octopart
Digi-Key
Mouser
Newark
Farnell
SparkFun
Adafruit
packages
DIP
SOT
SOIC
TSSOP
TQFP
LQFP
MLF, WLCSP, BGA
in-system development
ISP (original AVR)
software
avrdude
hardware
header
pads
clip
pins
Atmel-ICE
Arduino
ATmega16U2 Zaerc
ATtiny45 Sean
Alex
Tomás
Jonathan
Brian
Zaerc
ATtiny44 Ali
Valentin
Bas
Andy
David
USB-t44-ISP
board
components
traces
interior
firmware.zip
programming
UPDI (AVR 0,1,2-series,DA,DB,DD)
software
pyupdi
install:
pip3 install intelhex pylint pyserial
program:
python3 pyupdi.py -d your_processor -c your_port -b your_baud_rate -f your_hex_file -v
pymcuprog
install:
apt install libusb-1.0-0-dev, libudev-dev
pip3 install pymcuprog
program:
pymcuprog -d your_processor -t uart -u your_port -c your_baud_rate -v info erase
pymcuprog -d your_processor -t uart -u your_port -c your_baud_rate -v info write -f your_hex_file
hardware
USB-D11C-serial
board
components
top
interior
bootloader
SAMD11C
SAMD11C_serial
video
serial-UPDI-2 pin
board
components
traces
interior
connector(less)
serial UPDI-3 pin
board
components
traces
interior
USB-FT230X-UPDI
board
components
traces
interior
JTAG SWD (ARM)
software
OpenOCD
EDBG
program: edbg -b -t target_type -epv -f binary_file
read fuses: edbg -b -t target_type -F r,*,file_name
write fuses: edbg -b -t target_type -F w,high_bit:low_bit,bit_value
disable SAMD bootloader protection: edbg -b -t target_type -F w,2:0,7
hardware
Atmel-ICE
PICO
GoodFET
Raspberry Pi
Free-DAP
USB-D11C-SWD-10 pin
board
components
traces
interior
USB-D11C-SWD-4 pin
board
components
traces
interior
SWD-10-4-0.05 pitch
board
components
traces
interior
SWD-10-4-0.1 pitch
board
components
traces
interior
binary
video
prorgammer voltage input
bootloader, bootloaders
breakout
assembly language
hex file
instruction set, opcodes
mnemonics, directives, expressions
avr-as
inline
C
C++
programming
GCC
make files (tabs)
bit operations
AVR
libc
modules
types
math
benchmarks
avr-libc
binutils-avr
gcc-avr
homebrew-avr
ARM
gcc-arm-none-eabi
gdb-multiarch
homebrew-arm
libopencm3
Microchip
ASF
Studio
toolchains
packs (zip)
IDE
Atmel Studio
Arduino
Eclipse
VS Code
Scratch
Modkit
Firefly
frameworks
Arduino
board + toolchain + libraries + IDE + bootloader + header
programming
C++
Create
Codebender
Ardublock
CLI
original
Fabkit
Fabio
satshakit
hello.328P
cores
ATtiny
ATTinyCore
tinyAVR
DxCore
fab-sam
ESP8266
ESP32
overhead
millis
interrupts
ISR
PlatformIO
Mbed
boards
Atmel
Adafruit
Olimex
Polulu
MattairTech
Adrianino
SAMDino
D11C devkit
D21E devkit
clocks
types
RC (10%, 1% calibrated)
ceramic (0.5%)
quartz (50 ppm)
PLL
instruction cycles
overclocking
underclocking
host communication
RS232
bit timing
VT100/ANSI/ISO/ECMA terminal
Screen
Kermit
Minicom
Arduino
pySerial
miniterm
python -m serial.tools.miniterm /dev/your_device_port your_baud_rate
SerialPort
terminal
serialport-terminal
Web Serial
USB
software
V-USB
hardware
LUFA
ASF
Arduino
USB-D11C-serial
board
components
top
interior
bootloader
serial.ino
video
SAMD11C_serial
FTDI
drivers
libFTDI
cable
chip
hello.USB-serial.FT230X
board
components
traces
interior
ATtiny412
t412-blink-3 pin
board
components
traces
interior
blink.ino
blink.c
make
video
t412-blink-2 pin
board
components
traces
interior
blink.ino
video
ring.ino
t412-echo
board
componenets
traces
interior
echo.ino
video
echo.c
echo.make
video
ATtiny45
t45-echo
board
components
traces
interior pin re-use
ports
port.c
port.make
port.ino
port.write.ino
bit-bang
echo.c
echo.make
echo.ino
echo.serial.ino
video
ATtiny1614
ATtiny1624
t1614-echo
board
components
traces
interior
echo.ino
video
ATtiny44
t44-echo
board
components
traces
interior
t44-echo-2 sided
board
components
top
bottom
holes
interior
programming
mods
echo.c
echo.c.make
echo.interrupt.c
echo.interrupt.c.make
echo.asm
echo.asm.make
ATtiny3216
ATtiny3226
t3216-echo
board
components
traces
interior
echo.ino
video
AVR128DB32
128DB32-echo
board
components
traces
interior
ring.c
ring.make
echo.c
echo.make
video
ATSAMD11C
D11C-blink
board
components
traces
interior
D11C-blink-reset
board
components
traces
interior
D11C-blink-reset-clock
board
components
traces
interior
blink.c
blink.make
bootloader
blink.ino
video
ring.ino
D11C-echo-4 pin
board
components
traces
interior
D11C-echo-10 pin
board
components
traces
interior
D11C-echo-micro
board
components
traces
holes
interior
echo.ino
video
D11C-serial-1 sided
board
components
top
interior
D11C-serial-micro-4 pin
board
components
traces
holes
interior
Gerber
D11C-serial-large vias
board
components
top
bottom
holes
interior
D11C-serial-small vias
board
components
top
bottom
holes
interior
Gerber
serial.ino
video
SAMD11C_serial
ATSAMD11D
D11D-echo
board
components
traces
interior
openocd.cfg
bootloader
echo.ino
video
ATSAMD21E
D21E-echo
board
components
traces
interior
bootloader
echo.ino
video
Python
ATSAMD51
hello worlds
ring.ino
Interpreters
AVRSH
BASIC
FORTH
Espruino
MicroPython
CircuitPython
ESP8266
ESP-01 module
ESP-01-echo
board
components
traces
holes
interior
echo.ino
video
ESP-WROOM-02D module
ESP-WROOM-02D-echo
board
components
traces
holes
interior
echo.ino
video
Python
video
ESP32
ESP32-WROOM-32 module
ESP32-WROOM-echo
board
components
traces
holes
interior
echo.ino
video
ring.ino
Python
video
Operating Systems
FreeRTOS
TinyOS
Mbed OS
ROS
Systems
Rasberry Pi
ring.bcm2835.c
Beagle
Micro:bit
debugging
hardware
inspect, reflow solder joints
check component orientation, values
verify data sheets
confirm connector orientation
measure supply voltages
probe I/O signals
software
blink LED
add print statements
use embedded debugger
gdb, ddd
Atmel Studio
assignment
individual assignment:
read the data sheet for your microcontroller
use your programmer to program your board to do something
extra credit: try other programming languages and development environments
group assignment:
compare the performance and development workflows for other architectures